Handling PDFs is an integral part of many workflows, from business documents to educational resources. Sometimes, PDFs contain a hierarchical structure known as nested bookmarks, which organize the document into sections and subsections. If you’re looking to split a PDF into smaller files based on these nested bookmarks, Python’s PyPDF2 library is one of the best tools for the job. In this article, we’ll guide you through using PyPDF2 to split PDFs by nested bookmarks, explaining each step in simple terms so even a beginner can follow along.
What Are Nested Bookmarks in a PDF?
Nested bookmarks in a PDF act as an interactive table of contents. They allow users to navigate through a document quickly by grouping related sections and subsections in a hierarchical structure. Think of them as the digital equivalent of a book’s table of contents—except they are clickable and instantly take you to the desired page.
For instance, in an academic paper or eBook, you may find a top-level bookmark called “Chapter 1,” and under it, sub-bookmarks like “Introduction,” “Methods,” and “Conclusion.” These bookmarks improve user navigation and enhance the readability of a document, especially in longer files.
Why Do PDFs Have Bookmarks?
PDF bookmarks are not just decorative; they serve several practical purposes:
- Improved Navigation: In lengthy documents, bookmarks allow users to jump to specific sections without scrolling endlessly.
- Professional Presentation: PDFs with bookmarks appear organized and professional, which is crucial for business reports or presentations.
- Enhanced Usability: Bookmarks make it easier for users to locate content, saving time and effort.
- Hierarchical Organization: For documents like books, manuals, or multi-section reports, bookmarks enable a structured hierarchy that reflects the document’s layout.
When dealing with PDFs that have nested bookmarks, you can extract specific sections, split the document, or organize its content efficiently using Python.
How Nested Bookmarks Work in PDFs
Nested bookmarks use a hierarchical structure to organize content. The hierarchy is composed of parent and child bookmarks, where a parent bookmark represents a primary section, and child bookmarks represent subsections or related content within the parent category.
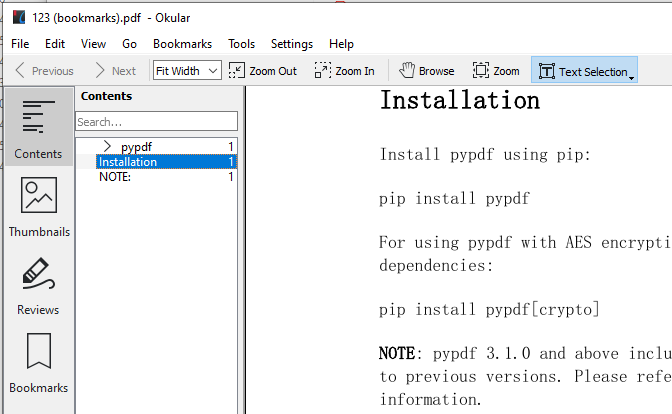
Examples of Nested Bookmarks in Real PDFs
To better understand nested bookmarks, here are some real-life examples:
- Technical Manuals:
- Parent: Chapter 1 – Introduction
- Child 1: What Is This Manual About?
- Child 2: How to Use This Manual
- Legal Documents:
- Parent: Article I – Definitions
- Child 1: Section A – Terms
- Child 2: Section B – Clauses
- eBooks:
- Parent: Chapter 5 – Data Science
- Child 1: Python for Data Science
- Child 2: Machine Learning Basics
This hierarchy allows users to jump between sections and subsections quickly and logically.
What Is PyPDF2, and Why Use It?
PyPDF2 is a Python library designed for handling PDF files programmatically. With PyPDF2, you can extract text, merge or split documents, add watermarks, and manipulate PDFs in many ways. The library is lightweight, open-source, and works across platforms, making it an excellent choice for automating PDF-related tasks.
Features of PyPDF2
Some of the key features of PyPDF2 include:
- Merging PDFs: Combine multiple PDFs into a single document.
- Splitting PDFs: Extract specific pages or sections from a PDF.
- Reading and Writing Metadata: Access and modify information like the author, title, and subject.
- Encryption and Decryption: Add or remove password protection from PDFs.
- Bookmark Management: Access and manipulate bookmarks, which is especially useful for splitting by nested bookmarks.
Compared to many paid tools, PyPDF2 offers similar functionality for free, with the added flexibility of Python coding.
PyPDF2 vs Other PDF Tools
While there are other tools available for working with PDFs, PyPDF2 stands out for several reasons:
- Cost-Effective: PyPDF2 is free and open-source, whereas many other PDF tools require a paid subscription.
- Customizability: With Python, you can write custom scripts tailored to your specific needs, offering more flexibility than pre-built software.
- Automation: PyPDF2 enables batch processing of PDFs, saving time when dealing with large volumes of documents.
- Cross-Platform: It works on Windows, Mac, and Linux systems, making it accessible to a broad audience.
However, other tools like Adobe Acrobat Pro or PDFsam might be more user-friendly for non-technical users who are uncomfortable with coding.
Step-by-Step Guide to Splitting PDFs by Nested Bookmarks
Now that you understand the basics of PyPDF2 and nested bookmarks, let’s dive into the step-by-step process of splitting a PDF based on its bookmarks.
Install PyPDF2 on Your Computer
Before using PyPDF2, you need to install it. PyPDF2 can be installed easily using Python’s package manager, pip. Open your terminal or command prompt and run the following command:

bash
CopyEdit
pip install pypdf2
Once installed, you’re ready to write Python scripts that manipulate PDFs.
Write Python Code to Extract Nested Bookmarks
To split a PDF based on nested bookmarks, you’ll need to write a Python script. Below is a sample script to guide you:
python
CopyEdit
from PyPDF2 import PdfReader, PdfWriter
def split_pdf_by_bookmarks(input_file):
reader = PdfReader(input_file)
writer = PdfWriter()
def extract_bookmarks(bookmark, parent_title=””):
if isinstance(bookmark, list):
for child in bookmark:
extract_bookmarks(child, parent_title)
else:
title = parent_title + ” – ” + bookmark.title if parent_title else bookmark.title
writer.add_page(reader.pages[bookmark.page])
with open(f”{title}.pdf”, “wb”) as output_file:
writer.write(output_file)
writer.pages.clear()
extract_bookmarks(reader.get_outline())
# Example usage:
split_pdf_by_bookmarks(“sample.pdf”)
This script reads the input PDF, extracts its bookmarks, and splits the PDF into separate files based on those bookmarks.
Save Split PDFs with Bookmark Names
When splitting the PDF, the output files should ideally be named based on the bookmarks. This makes it easier to identify each section later. The script above automatically names the files using the bookmark titles. For example, if the bookmark says “Chapter 1,” the split file will be named Chapter 1.pdf.
Common Errors When Splitting PDFs with PyPDF2
While PyPDF2 is powerful, you may encounter some common issues:
- Encoding Errors: Bookmark titles with special characters may cause encoding errors. To fix this, ensure proper string encoding in your script.
- Corrupted PDFs: If the input PDF is corrupted or improperly formatted, PyPDF2 may fail to read the file.
- Complex Bookmarks: Some PDFs have deeply nested bookmarks or unconventional structures, which might require additional code to handle.
For troubleshooting these errors, carefully review the PDF structure and refine your script accordingly.
How to Debug Code Errors
Debugging Python scripts is an essential skill, especially when working with libraries like PyPDF2. If your code isn’t working as expected, follow these tips:
- Use Print Statements: Add print statements to verify the values of variables and bookmark structures.
- Check Documentation: Refer to the official PyPDF2 documentation or forums for guidance.
- Test with Different PDFs: Some PDFs may have unique structures. Test your code with a variety of files to identify edge cases.
The Bottom Line
Splitting PDFs by nested bookmarks using PyPDF2 is a practical and efficient way to handle complex documents. Whether you’re working with technical manuals, eBooks, or legal documents, PyPDF2 gives you the tools to automate and simplify your workflow. By following this guide, you can leverage the power of Python to process PDFs with ease.
With its flexibility, cost-effectiveness, and wide range of features, PyPDF2 is a fantastic choice for programmers and non-programmers alike. So, install PyPDF2 today, and start organizing your PDFs with precision and efficiency!
Leave a Reply